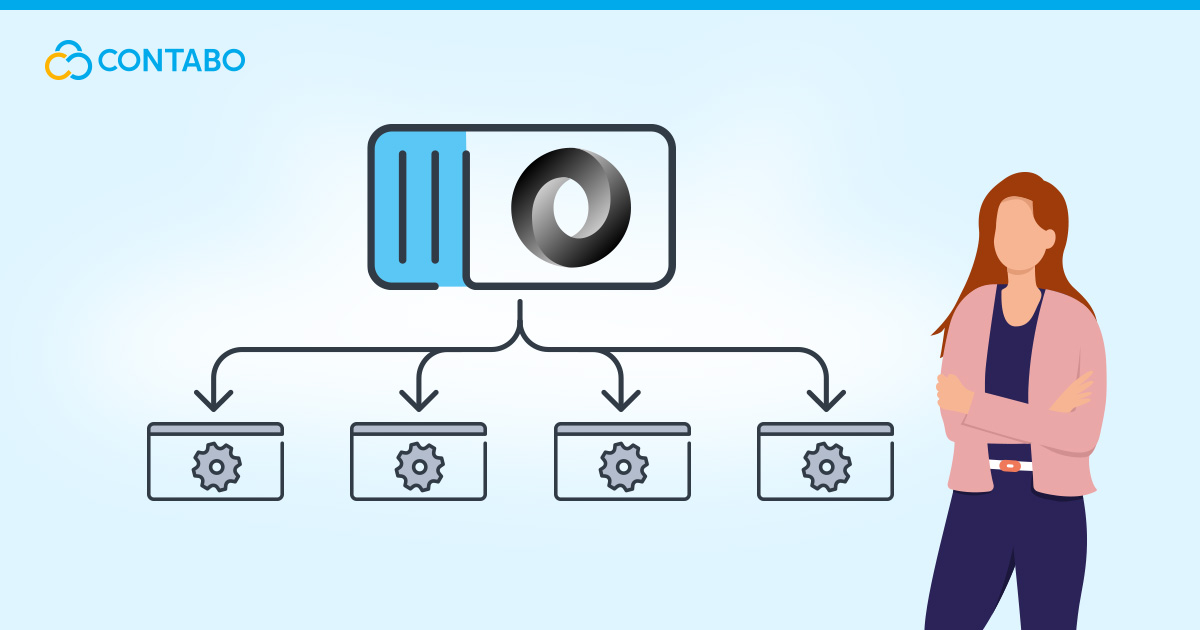
This tutorial offers a straightforward solution for developers that want to set up mock APIs quickly with JSON Server. It simplifies the creation of a fake REST API, allowing for immediate testing and development of frontend applications without the wait for a backend to be built. This tool is particularly useful for prototyping, testing data interactions, and speeding up the development cycle by focusing on frontend needs. By using JSON Server, developers can easily simulate backend services with just a JSON file, making it an efficient way to develop, test, and refine applications. This guide will walk you through the process, helping you learn how to install JSON Server and use it to create mock APIs, ensuring you make the most of this tool in your development projects.
What is a JSON Server?
A JSON Server is a nimble tool enabling developers to swiftly establish a mock REST API. It leverages a simple JSON file to emulate the data output one would expect from a genuine API, thus functioning as a temporary backend during the development phase. This setup facilitates direct data interaction through HTTP requests, effectively simulating a real web server’s operations without the intricacies of configuring databases and server-side programming.
Purpose of a JSON Server
The main goal of using a JSON Server is to boost frontend development efficiency. It provides developers with a dependable, easily adjustable backend substitute, allowing them to concentrate on crafting and refining the user interface. This utility is particularly important during the initial stages of project development or when quick prototyping is essential, offering a seamless way to proceed without the actual backend.
Typical Use-Cases of JSON Servers
JSON Servers find their place in a variety of scenarios, most notably in prototyping new features, testing frontend designs, and building standalone applications that demand minimal backend interaction. They also serve an educational purpose, offering a hands-on experience for those new to development by demonstrating how frontends communicate with backends in a simplified environment. By mimicking real data transactions, JSON Server streamlines the development workflow, proving to be an invaluable asset for developers aiming to enhance efficiency and focus on delivering user-oriented applications.
Advantages of Using JSON Server
Speed and Simplicity in API Mocking
JSON Server stands out for its ability to quickly set up mock APIs, a process that is both fast and straightforward. Developers can generate a fully functional REST API based on a simple JSON file in minutes, bypassing the complexity typically associated with backend development. This rapid setup is ideal for testing, prototyping, and any scenario where time is of the essence.
Flexibility in Testing Frontend Applications
The flexibility JSON Server offers in testing frontend applications is among the most appreciated features. It allows developers to easily modify data structures, simulate server responses, and test various scenarios without altering a real backend. This adaptability makes it easier to identify and address frontend issues early in the development process, ensuring a smoother, more efficient path to a polished final product.
Frameworks and JSON Server
Compatibility with React, Angular, Vue.js, and More
JSON Server’s adaptability is a key asset, offering seamless compatibility with a wide array of frontend frameworks, including React, Angular, and Vue.js. Its flexibility doesn’t stop there; it extends to virtually any framework or library capable of making HTTP requests. This broad compatibility ensures developers can integrate mock APIs into their projects, no matter their preferred technology stack.
Enhancing Development with Framework-Specific Integrations
Leveraging JSON Server across different frameworks can significantly elevate the development experience. React developers, for example, can swiftly prototype with state management, Angular enthusiasts can mock services for HTTP client tests, and Vue.js experts can enhance data handling and component testing. This integration facilitates a more efficient development process, enabling tailored testing and debugging strategies that align with the unique demands of each framework.
The Value of Fake or Mock APIs
Accelerating Development Cycles
Utilizing fake or mock APIs, such as those created with JSON Server, significantly accelerates the development process. By providing a stand-in for backend services, developers can proceed with frontend tasks without waiting for the backend to be fully implemented. This parallel development approach reduces the overall project timeline, enabling faster iterations and quicker feedback loops.
Simplifying Testing and Debugging
Mock APIs simplify the testing and debugging phases of development. They allow for the isolation of frontend functionality, making it easier to pinpoint issues directly related to the user interface or data handling. This clarity is invaluable for efficient problem-solving and ensures that when the real backend is integrated, the frontend is already polished and robust. Moreover, the ability to simulate various backend responses with mock APIs aids in preparing the frontend for a wide range of real-world scenarios.
JSON Server Installation Prerequisites
System Requirements of a JSON Server
To set up JSON Server, you need a basic development environment with Node.js installed. This setup ensures you can run the npm (Node Package Manager) commands required for installation. JSON Server is lightweight, making it compatible with most operating systems that support Node.js, including Windows, macOS, and Linux.
JSON Server Hosting Recommendations
For development and testing purposes, running JSON Server on your local machine is usually sufficient. However, if you’re considering a more persistent setup or need to share your mock API with a team, deploying it on a Virtual Private Server (VPS) or a Virtual Dedicated Server (VDS) is recommended. These options offer a balance between cost, performance, and isolation, making them ideal for development environments. A Dedicated Server might be more than necessary for JSON Server’s modest requirements, unless you’re also hosting other services or applications that demand significant resources.
Setting Up a JSON Server
Setting up a JSON Server on a Debian-based Linux distribution is a straightforward process that involves a few simple steps. This setup allows developers to quickly simulate a backend REST API using a single JSON file.
Overview of Installation Steps
The installation process involves installing Node.js, setting up JSON Server, and then configuring it to suit your project’s needs. Here’s how to get started:
Commands for Setup
Install Node.js
First, ensure Node.js is installed on your system. If it’s not, you can install it using the following commands:
sudo apt update
sudo apt install nodejs npm
This installs both Node.js and npm, which is the package manager for Node.js.
npm Install
With Node.js and npm installed, you can now install JSON Server globally on your machine. This allows you to use it from any directory.
sudo npm install -g json-server
Starting the JSON Server
To start the JSON Server, navigate to the directory where your db.json file is located (or where you plan to create it) and run:
json-server --watch db.json
This command starts the server and watches the db.json file for changes, automatically updating the data served by the API.
Changing the Ports of a JSON Server
By default, JSON Server runs on port 3000. If you need to use a different port, you can specify it with the –port flag:
json-server --watch db.json --port 8000
Additional Configuration Options
JSON Server offers various flags for customization, such as serving static files, enabling CORS, and more. For example, to serve static files from a public directory alongside your API, you can use:
json-server --watch db.json --static ./public
By following these steps, you’ll have a fully functional mock API server running on your Debian-based system, ready for development and testing.
Implementing CRUD Operations and Custom Routes
Basics of CRUD on JSON Server
JSON Server provides a full-fledged simulation of CRUD (Create, Read, Update, Delete) operations, making it an excellent tool for frontend development. Once your server is up with a db.json file, you can easily perform these operations over HTTP requests:
- Create: To add new data, use POST requests. For example, POST /posts adds a new post to the posts collection in your db.json.
- Read: Retrieve data with GET requests. A simple GET /posts fetches all posts, while GET /posts/1 fetches the post with an ID of 1.
- Update: PATCH or PUT requests update existing data. For instance, PATCH /posts/1 updates partial data of the post with ID 1.
- Delete: Remove data using DELETE requests, like DELETE /posts/1 to delete the post with ID 1.
Defining and Using Custom Routes
Beyond basic CRUD, JSON Server allows for defining custom routes in a routes.json file, offering more flexibility in how you structure your API endpoints. This feature lets you create more meaningful and specific endpoints for your application needs. For example:
{
"/api/posts/:id": "/posts/:id"
}
This route definition tells JSON Server to treat requests to /api/posts/:id as if they were made to /posts/:id, allowing for a more intuitive API structure. To use custom routes, start the server with the –routes flag:
json-server db.json --routes routes.json
Implementing CRUD operations and custom routes with JSON Server not only simplifies backend simulation but also enhances your application’s development by providing realistic data interaction and API structure.
Advanced Features of a JSON Server
JSON Server offers a suite of advanced features that extend its functionality beyond basic CRUD operations, enabling more sophisticated simulations of real-world API behavior.
Sorting, Searching, and Pagination
- Sorting: You can sort the data returned by your API by appending _sort and _order parameters to your queries. For example, GET /posts?_sort=title&_order=asc sorts posts by their titles in ascending order.
- Searching: Implement search functionality by using the q parameter. A query like GET /posts?q=javascript searches for “javascript” within the posts.
- Pagination: JSON Server supports pagination out of the box. Use _page and _limit parameters to paginate the data, such as GET /posts?_page=2&_limit=10 to get the second page of posts, limiting to 10 posts per page.
Parsing Data Correctly
Ensuring your data is parsed correctly is important for the accurate functioning of your API. JSON Server automatically handles JSON data parsing, but when dealing with more complex data structures or when you need to enforce specific data validations, you might consider using middleware to parse and validate incoming data before it’s processed.
Advanced Features in Detail
- Creating Middleware for Authentication and Logging: Enhance your JSON Server with custom middleware to simulate authentication mechanisms or to log requests and responses. Middleware functions can intercept requests to perform checks or log data, adding a layer of realism to your mock API.
- Serving Static Files: JSON Server can also serve static files. Place your static assets in a public directory and start the server with the –static flag to serve these files alongside your mock API, allowing you to test frontend assets in a more integrated environment.
- Simulating Slower Network Conditions: To better understand how your application behaves under different network conditions, you can introduce artificial delays in your responses using middleware. This simulation helps in optimizing frontend performance and improving user experience under constrained conditions.
By leveraging these advanced features, developers can create a more realistic and controlled development environment, allowing for thorough testing and refinement of applications before they interact with real backend services.
JSON Server Best Practices for Error Handling
Managing Errors and Unexpected Responses
Effective error handling is essential for maintaining a reliable API. JSON Server allows you to simulate error responses, enabling you to test how your application reacts to various failure scenarios. Implementing custom middleware can help manage errors by intercepting requests and generating appropriate error responses. For example, checking for missing fields in a POST request and returning a 400 Bad Request status if necessary. This practice helps in identifying potential issues that could disrupt the user experience.
Ensuring Robustness in API Interaction
To ensure robustness in API interactions, it’s important to anticipate and gracefully handle errors on the frontend. This includes implementing checks for HTTP status codes, parsing error messages correctly, and providing clear feedback to users. Testing your application with JSON Server under different error conditions allows you to refine these error-handling mechanisms, ensuring that your application remains functional and user-friendly, even when unexpected issues arise.
Security Considerations for JSON Servers
Understanding the Security Implications
Using JSON Server, especially in a shared or public setting, introduces security considerations that also touch on privacy concerns. Since it’s designed for development purposes, JSON Server doesn’t include built-in authentication or encryption, which means data and interactions could be exposed to unauthorized users, potentially compromising privacy. Recognizing these limitations is crucial for safeguarding your mock APIs, the data they handle, and the privacy of any data subjects involved.
Mitigating Risks Associated with Mock APIs
To mitigate security and privacy risks, it’s advisable to run JSON Server in a controlled environment. This could be locally on your development machine or within a secure, private network. If you need to share your API with a wider team, consider using VPNs or access control mechanisms to restrict access, as this approach enhances privacy protection. Additionally, avoid using sensitive real-world data in your mock APIs to help prevent privacy breaches. For more secure and privacy-conscious mock API deployments, explore tools and platforms, and look for those offering enhanced security features. These include authentication and HTTPS support, which help to protect your data and interactions and ensure the privacy of individuals represented in your data.
Alternatives to JSON Server
While JSON Server is a popular choice for setting up mock APIs, several other tools and services are used by the community that offer similar functionalities with their own unique features:
- Mirage JS (Mirage JS): A client-side server to develop, test, and prototype your app. Ideal for frontend developers needing full control over API responses.
- Mockoon (Mockoon): Offers a user-friendly interface for creating mock APIs locally. It supports environment simulation and route configuration without coding.
- WireMock (WireMock): A flexible library for stubbing and mocking web services. It has extensive request matching and response templating capabilities, suitable for more complex scenarios.
- Postman Mock Servers (Postman): Part of the Postman API platform, allowing users to create and manage mock servers directly within their API testing suite.
These alternatives provide a range of options depending on your project’s needs, from simple mock setups to more detailed API simulations and testing environments.
Conclusion
Throughout this guide, we’ve explored how JSON Server facilitates rapid development by allowing for the easy setup of mock REST APIs, enabling CRUD operations, and supporting advanced features like sorting, searching, and custom routes. Its compatibility with various frontend frameworks and the ability to simulate real-world scenarios make it an invaluable tool for developers. While mindful of security considerations, JSON Server’s simplicity and flexibility in testing and prototyping are unmatched. Using JSON Server in your development workflow can significantly streamline the process, making it easier to focus on creating robust, user-centric applications. As we’ve seen, alternatives exist, but JSON Server remains a standout choice for its ease of use and comprehensive feature set, empowering developers to achieve more in less time.