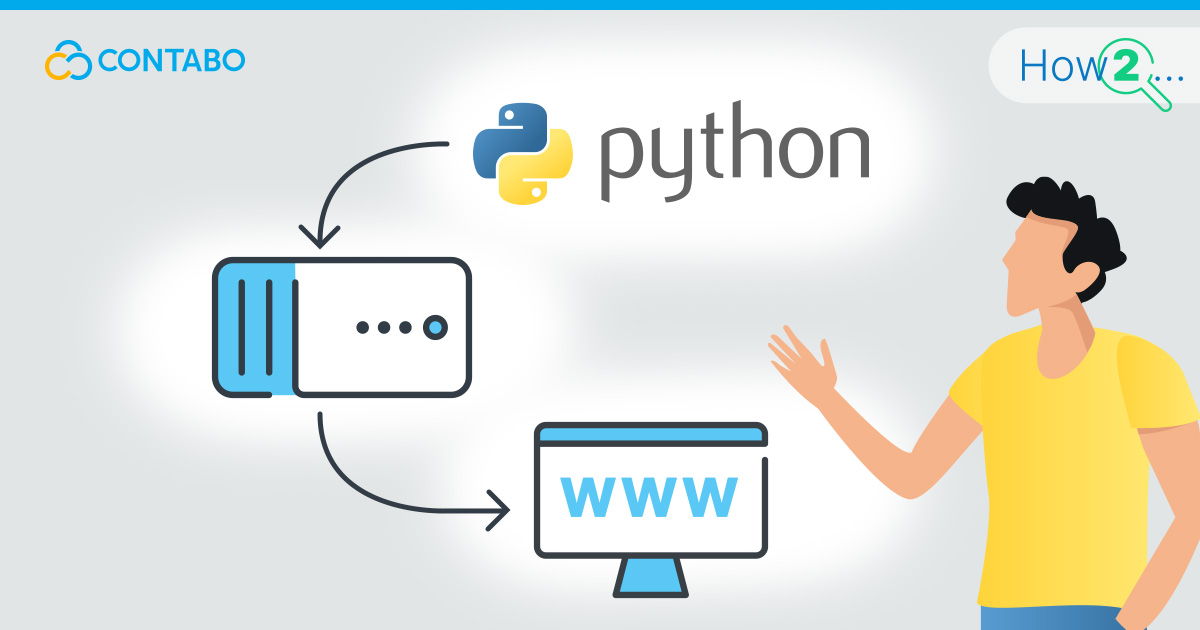
This guide is designed to help software developers quickly start a local python web server for testing, namely set up a local web server using Python, a powerful and versatile programming language. Whether you are developing a new web application or testing an existing one, understanding how to run a local server is always the first step. Our objective is to provide you with practical steps to use Python for serving web content directly to your browser. You will learn how to write simple Python code to manage web requests, serve files, and test your applications in a local development environment. By the end of this tutorial, you will have the knowledge to efficiently run and test your web projects on a Python-based server, using tools and commands that streamline your development workflow. Let us get started and explore how to leverage Python for local web server testing.
Understanding Python Web Servers
Definition and Use-Cases
A Python web server is a software system designed to process web requests using the HTTP or HTTPS protocols and deliver web content to clients. These servers are widely used by developers for various purposes, including testing web applications in a local environment, developing small to medium-scale web applications, and serving dynamic content with frameworks like Flask or Django. The flexibility and ease of scripting server behavior make Python web servers an excellent choice for rapid development cycles, allowing for quick iterations and immediate feedback during the testing phase.
Comparison with Apache and NGINX
When it comes to choosing a web server, the decision often hinges on the project’s specific requirements. Here is a brief comparison of Python web servers with traditional options like Apache and NGINX:
Feature | Python Web Server | Apache | NGINX |
Ease of Setup | Easy to set up with minimal configuration required. | Involves more complex setup and configuration. | Similar to Apache but optimized for better performance. |
Flexibility | Highly flexible, ideal for development, testing, and lightweight applications. | Highly configurable, suitable for a wide range of production environments. | Offers high efficiency, designed for handling high concurrency scenarios. |
Performance | Best suited for development and small-scale applications. | Optimized for high performance, capable of scaling for large-scale applications. | Known for excellent performance, especially in handling high traffic loads efficiently. |
Use Case | Perfect for testing, development, and serving dynamic content in a controlled environment. | Preferred for hosting large-scale web applications and complex websites. | Ideal for high-traffic websites, load balancing, and as a reverse proxy. |
Python web servers shine in development and testing environments due to their simplicity and ease of use. However, for production environments dealing with high traffic and requiring robust performance, traditional servers like Apache and NGINX are more suitable. The choice between these servers should be based on the scale of the project, performance requirements, and specific server features needed.
Prerequisites for Starting a Local Python Web Server
To begin setting up a local Python web server, you will need to ensure you have the following essentials:
Python Installation: Make sure you have Python 3.x installed on your machine for access to the latest features and support. For Debian-based distributions, you can install Python by running the following commands in your terminal:
sudo apt update
sudo apt install python3
Text Editor: A reliable text editor or Integrated Development Environment (IDE) is important for coding. Consider using Visual Studio Code, PyCharm, or Sublime Text for writing and editing your Python scripts.
Basic Python Knowledge: A fundamental understanding of Python syntax and core programming concepts is required. Being able to write and execute Python scripts is key to successfully setting up your web server.
Command Line Usage: Familiarity with the command line or terminal is essential for running commands, navigating through directories, and managing files. If you are new to the Linux command line, our article “Linux Command Line – Tips and Tricks” offers a great starting point to learn the basics.
Web Development Basics: Although not strictly necessary, knowing the basics of web development, including how HTTP requests and responses work, will prove beneficial.
With these tools and knowledge at your disposal, you are ready to proceed with setting up your local Python web server.
Python Frameworks for Web Development
Python offers several frameworks that simplify web development, enabling you to create robust web applications efficiently. Flask and Django are two of the most popular choices among developers.
Flask is a lightweight framework that provides the essentials to build web applications quickly. It is designed to be simple and extensible, making it ideal for small to medium projects. Flask allows you to start with simple, single-file applications and scale up to complex applications.
Django is a high-level framework that encourages rapid development and clean, pragmatic design. It includes an ORM (Object-Relational Mapping), which abstracts database operations, and comes with a built-in admin panel. Django is suited for larger applications with its “batteries-included” approach.
Other notable frameworks include Pyramid, which sits between Flask and Django in terms of features, and Tornado, known for its asynchronous capabilities and ability to handle long-lived network connections.
Choosing the right framework depends on your project’s requirements, your familiarity with the framework, and the specific features you need.
Setting Up Your Local Python Web Server
Setting up a local Python web server involves a few straightforward steps, from installing Python to setting up your project directory and choosing a web framework like Flask. Here is how to get started across different operating systems:
Installing Python
Windows, macOS, and Linux users can download Python from the official Python website. For Linux users, particularly those on Debian-based distributions, Python can be installed using the terminal with the commands mentioned earlier. Ensure you have Python 3.x installed by running python3 –version in your terminal or command prompt to check your Python version.
Installing Flask
Flask can be installed using pip, Python’s package installer. Open your terminal or command prompt and run the following command to install Flask:
pip install Flask
This command works across Windows, macOS, and Linux, provided you have Python and pip installed.
Setting Up a Project Directory
- Create a Project Directory: Choose a location on your computer where you want to store your project files. Create a new directory by running mkdir my_project in your terminal or command prompt, then navigate into it with cd my_project.
- Initialize a Virtual Environment (optional but recommended): A virtual environment allows you to manage dependencies for your project separately. Create one by running python3 -m venv venv. Activate it with source venv/bin/activate on macOS/Linux or .\venv\Scripts\activate on Windows.
- Installing a Web Framework: With your virtual environment activated, install Flask (or your chosen framework) using pip if you have not already.
- Create Your First App: Inside your project directory, create a file named app.py. Open this file in your text editor or IDE and write a simple Flask application:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
- Run Your Server: With your Flask app written, go back to your terminal or command prompt, ensure you are in your project directory, and run python app.py. This starts your local web server.
- Access Your Server: Open your web browser and navigate to http://localhost:5000. You should see “Hello, World!” displayed, indicating your local Python web server is running successfully.
By following these steps, you have successfully set up a local Python web server using Flask, ready for development and testing of web applications.
Developing Your First Web Application
Creating your first web application with Python involves handling both static and dynamic content. Static content includes files like images, CSS, and JavaScript that do not change, while dynamic content is generated on-the-fly by the server based on user requests. Here is a simple guide to get you started:
Handling Static Content
- Organize Static Files: Create a folder named static in your project directory. Place your static files, such as images, CSS, and JavaScript files, in this folder.
- Serve Static Files: Flask automatically serves static files from the static folder. Access these files in your HTML by using the URL /static/filename. For example, if you have an image named logo.png in the static folder, you can include it in your HTML with <img src=”/static/logo.png”>.
Handling Dynamic Content
- Create Templates: Dynamic content is typically served through templates. Create a folder named templates in your project directory. Inside this folder, create an HTML file, such as index.html, to serve as your template.
- Render Templates: Use Flask’s render_template function to serve your HTML file. Modify your app.py to include:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
This code tells Flask to render the index.html template when the root URL is accessed.
Running and Accessing the Web Server
- Start the Server: Run your Flask application by executing python app.py in your terminal. This starts the web server.
- Access the Application: Open a browser and go to http://localhost:5000. You will see your web application running. The root route (/) will display the index.html template, showcasing how Flask handles both static and dynamic content.
By following these steps, you have created a basic web application that serves both static and dynamic content. Experiment with different routes, templates, and static files to further explore Flask’s capabilities.
Database Integration
Integrating a database into your Python web server enables dynamic content management and storage, essential for most web applications. Python supports several types of databases, from lightweight options like SQLite to more robust systems such as PostgreSQL and MySQL. Here is a brief overview of connecting to databases and some recommendations:
Connecting to Databases
- Choose a Database: Select a database that fits your project’s needs. SQLite is ideal for development and small applications due to its simplicity and ease of setup. For production environments or applications requiring more scalability, consider PostgreSQL or MySQL.
- Use an ORM: An Object-Relational Mapping (ORM) tool like SQLAlchemy or Django’s built-in ORM simplifies database operations. ORMs allow you to interact with your database using Python code instead of SQL queries, making your code more maintainable and portable.
- Establish a Connection: Install the necessary database driver for your chosen database (e.g., psycopg2 for PostgreSQL). Then, configure your web application to connect to the database using the ORM’s connection settings. For example, in Flask with SQLAlchemy:
from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] =
'postgresql://username:password@localhost/mydatabase'
db = SQLAlchemy(app)
Recommended Types for Python Web Servers
- SQLite: Best for development and testing due to its simplicity and zero configuration.
- PostgreSQL: PostgreSQL Highly recommended for production environments requiring robust features, data integrity, and scalability.
- MySQL: MySQL Another popular choice for web applications, known for its reliability and performance.
Choosing the right database depends on your application’s size, complexity, and specific requirements. Integrating a database with your Python web server is straightforward with the right tools and libraries, enabling you to build dynamic, data-driven web applications.
Security Considerations
Ensuring the security of your local Python web server is essential, even during development. Here are some basic security measures to implement:
- Update Regularly: Keep Python, your web framework, and all dependencies up to date to protect against known vulnerabilities.
- Use HTTPS: Even for local testing, consider using HTTPS to encrypt data in transit. Tools like Let Us Encrypt provide free SSL/TLS certificates, or you can use self-signed certificates for development purposes.
- Validate Input: Always validate user input to prevent common web vulnerabilities such as SQL injection and cross-site scripting (XSS). Use built-in framework features or libraries designed for input validation.
- Limit Access: If your local server is accessible on a network, restrict access using firewall rules or by binding the server to localhost (127.0.0.1) to prevent unauthorized external access.
- Use Environment Variables: Store sensitive information like database credentials in environment variables instead of hardcoding them into your application.
By following these basic security practices, you can significantly reduce the risk of vulnerabilities in your local web server setup.
Practical Example Project
Let us create a simple yet functional Python web application using Flask that displays a greeting and the current time. This project will demonstrate handling dynamic content and give you a hands-on experience with Flask.
Step 1: Set Up Your Environment
- Ensure Python and Flask are installed.
- Create a new directory for your project, e.g., flask_time_app.
- Navigate into your project directory and activate a virtual environment.
Step 2: Create Your Flask Application
- Initialize Your App: In your project directory, create a file named app.py. Open this file in your text editor and add the following code:
from flask import Flask
from datetime import datetime
app = Flask(__name__)
@app.route('/')
def home():
current_time = datetime.now().strftime('%H:%M:%S')
return f'Hello, the current time is {current_time}'
if __name__ == '__main__':
app.run(debug=True)
This code initializes a Flask application and defines a single route (/) that, when accessed, displays a greeting along with the current time.
Step 3: Run Your Application
- In your terminal, run the command python app.py to start your Flask server.
- Open a web browser and navigate to http://localhost:5000. You should see a message displaying “Hello, the current time is [current time].”
Step 4: Explore Further
- Try adding more routes to display different messages or content.
- Experiment with Flask templates to render HTML pages instead of plain text.
This simple project introduces you to developing web applications with Flask, handling dynamic content, and the basics of web server operation. As you become more comfortable, you can expand this project with more complex functionality, such as database integration or user input handling.
Common Challenges and Troubleshooting
Beginners often encounter a few common issues when setting up a Python web server:
- Server Not Starting: Ensure Python and Flask are correctly installed. Check for syntax errors in your app.py file.
- Port Already in Use: If you receive an error stating the port is already in use, change the port by modifying the app.run(debug=True, port=5001) command in your app.py.
- Static Files Not Loading: Verify that static files are placed in the correct static directory and referenced correctly in your HTML or Flask routes.
- Template Not Found: Ensure your HTML templates are stored in the templates folder and that you are using the correct filename in your render_template function.
For most issues, reviewing error messages and consulting Flask documentation can provide quick solutions. Additionally, ensure your development environment is set up correctly and that you are following Flask conventions for file and directory structures.
Exploring Alternatives
Beyond Python and Flask, several other technologies offer robust solutions for local web development:
Node.js with Express
- Pros: JavaScript for both client and server-side, making it a seamless choice for developers familiar with JavaScript. Express.js offers a minimalistic framework for building web applications quickly.
- Cons: Callbacks and asynchronous code can be challenging for beginners. Managing large-scale applications might require additional tools and frameworks.
PHP
- Pros: Widely used for web development, with extensive documentation and a large community. Easy to get started for small projects and embedded with HTML.
- Cons: Can become unwieldy for modern web applications without a framework. The language has inconsistencies and a mixed reputation for design patterns.
Docker + NGINX
- Pros: Docker containers encapsulate the environment, making projects portable and consistent across different machines. NGINX is highly efficient for static content and load balancing.
- Cons: Docker has a learning curve and requires understanding containerization principles. NGINX configuration can be complex for beginners.
Compared to Python for local development, these alternatives offer different paradigms and ecosystems. Python remains a strong contender for its readability, simplicity, and the vast array of libraries and frameworks available, making it suitable for both beginners and experienced developers.
Conclusion
Throughout this tutorial, we have explored the essentials of setting up a local Python web server for testing, from installing Python and Flask to developing a simple web application that handles both static and dynamic content. We have also covered database integration, basic security measures, and addressed common challenges faced by beginners. Additionally, we looked at alternative technologies like Node.js with Express, PHP, and Docker + NGINX, highlighting their pros and cons compared to Python. Armed with this knowledge, you are now equipped to develop and test your web applications locally, leveraging Python’s simplicity and flexibility for your projects.