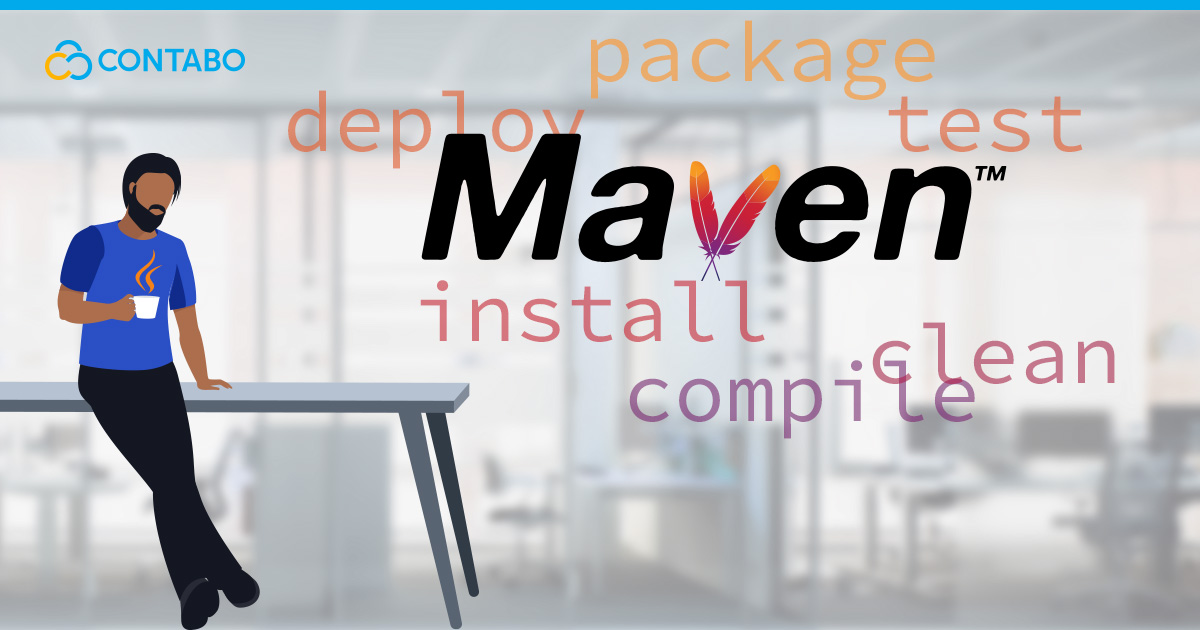
Maven commands are an essential Java development tool, streamlining the build process with efficiency and precision. Maven is more than just a build automation tool, acting like a comprehensive project management and comprehension tool. It simplifies the process of project builds, dependency management, and documentation, making it an indispensable asset for developers. Within Java projects, it ensures consistency, enhances project understanding, and facilitates team collaboration. Through the use of conventions for project layouts and an XML file to describe the project, Maven allows developers to manage builds, dependencies, and documentation with minimal effort. This cheat sheet aims to arm developers, from novices to seasoned Java professionals, with the knowledge to leverage Maven’s capabilities effectively, ensuring a smooth and efficient development process.
Installation and Setup
To start using Maven on Debian-based distributions, you will primarily use the terminal for installation and setup. Begin by updating your package index:
sudo apt update
Then, install Maven with the following command:
sudo apt install maven
This command downloads and installs Maven, along with its dependencies, onto your system. To confirm Maven has been installed successfully, execute:
mvn -version
This command displays the current version of Maven installed, verifying that Maven is ready for use. With Maven now installed, you are set to harness its capabilities for your Java projects, streamlining builds and managing dependencies with ease.
Basic Maven Commands
Maven simplifies Java project management through its powerful command-line interface (CLI). Here is a primer on essential Maven commands that every developer should know:
Creating a New Project
Use the mvn archetype:generate command to create a new project from an archetype template. This command prompts you to specify the groupId, artifactId, and version of your project, which are key identifiers in Maven’s convention. For example:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Compiling Your Project
Compile the source code of your project with mvn compile. This command compiles the source code and places the resulting .class files in the target/classes directory.
mvn compile
Running Tests
Execute mvn test to run the unit tests specified in your project using a suitable testing framework like JUnit. Maven automatically runs tests found in the src/test directory.
mvn test
Packaging the Project
To package your project into a JAR file, use mvn package. This command compiles your project and packages the compiled code along with any resources into a JAR file within the target directory.
mvn package
Cleaning the Project
Clean your project with mvn clean to remove the target directory, which contains all the build files, ensuring a fresh start for the next build.
mvn clean
Understanding and utilizing these basic Maven commands is foundational for Java developers, facilitating efficient project compilation, testing, and packaging.
Dependency Management
Maven excels in managing dependencies, automating the inclusion and management of libraries your project needs. Here is how to manage dependencies effectively:
Adding a Dependency
In your project’s pom.xml file, add dependencies by specifying their groupId, artifactId, and version within the <dependencies> tag. For instance, to add JUnit:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
Updating and Removing Dependencies
To update a dependency, change its version in the pom.xml. Removing a dependency simply requires deleting its <dependency> block from the pom.xml file.
Viewing Dependency Tree
To understand how dependencies are structured, use mvn dependency:tree. This command displays a tree of dependencies, showing which libraries your project directly or indirectly relies on.
mvn dependency:tree
By mastering dependency management, developers ensure that their projects use the correct library versions, avoiding conflicts and missing library issues, thereby maintaining the project’s health and stability.
Maven Lifecycle Phases
Maven’s lifecycle phases are a sequence of steps that define how to build and distribute a project. Understanding these phases is important for effectively managing and automating tasks within Maven projects. Here is an overview:
Overview of Lifecycle Phases
Maven organizes the build process into distinct phases, namely compile, test, package, and install. Each phase represents a stage in the build lifecycle, starting from validating the project, compiling source code, running tests, packaging the compiled code into a distributable format (like a JAR file), and finally installing the package into the local repository, which can then be used as a dependency in other projects.
Common Lifecycle Commands
Command | Description |
---|---|
mvn validate | Validates the project, checking if all necessary information is available. |
mvn compile | Compiles the project’s source code. |
mvn test | Runs the tests using a suitable unit testing framework, such as JUnit. |
mvn package | Packages the compiled code into a distributable format, such as a JAR. |
mvn verify | Runs any checks to verify the package is valid and meets quality criteria. |
mvn install | Installs the package into the local repository, making it available for use as a dependency in other projects. |
mvn deploy | Copies the final package to the remote repository for sharing with other developers and projects. |
Understanding and utilizing these lifecycle phases allows developers to automate and manage the build process efficiently, ensuring that the software development lifecycle is smooth and error-free.
Maven Plugins
Maven Plugins extend Maven’s capabilities, allowing developers to interact with and customize the build lifecycle. Here are some essential Maven plugins and their uses:
Understanding Maven Plugins
Plugins are the heart of Maven’s functionality, each designed to perform specific tasks within the build process. They are configured in the pom.xml file and are automatically invoked during relevant lifecycle phases.
Common Plugins and Their Uses
Compiler Plugin: The maven-compiler-plugin is used to compile the source code of your project.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
- Surefire Plugin for Testing: The maven-surefire-plugin is responsible for running tests. It is a powerful tool for executing and configuring unit tests.
- Javadoc Plugin: Generates Javadoc for your project, making it easier to understand and maintain the code.
- WAR Plugin for Web Applications: The maven-war-plugin is used for projects requiring WAR packaging, facilitating the creation of web application archives.
By leveraging these and other plugins, developers can enhance their Maven build process, tailoring it to their project’s specific needs while ensuring efficiency and consistency.
Advanced Maven Commands
As developers become more familiar with Maven, leveraging advanced commands can significantly optimize and customize the build process. Here are some powerful advanced Maven commands:
Skipping Tests: Sometimes, you may want to build your project without running tests to save time. Use the -DskipTests flag with your Maven command to skip the test phase.
mvn package -DskipTests
Setting Properties: Customize your build by setting project properties directly from the command line. This is particularly useful for passing dynamic values or configurations.
mvn install -Dproperty=value
Running Specific Goals: Execute specific goals for a plugin without going through the entire lifecycle. This command is handy for running a single action, such as generating a site or a report.
mvn <plugin>:<goal>
Debugging Maven: Encounter an issue? Use -X or –debug to print debugging output, providing insights into what Maven is doing behind the scenes. This information can be invaluable for troubleshooting.
mvn install -X
These commands allow developers to fine-tune the build process, addressing specific needs or challenges swiftly and effectively.
Maven Profiles
Maven Profiles offer a flexible way to customize build configurations for different environments, such as development, testing, or production. Profiles allow developers to define specific configurations that can be activated under certain conditions.
Introduction to Profiles
In your pom.xml, you can define profiles with specific build settings, dependencies, and plugins. Profiles can cater to various scenarios, like adjusting configurations for different deployment environments.
Activating Profiles
Activate a profile using the -P flag followed by the profile name when running Maven commands. This allows you to switch configurations seamlessly.
mvn package -Pproduction
By utilizing profiles, developers can easily manage and switch between different build configurations, ensuring that the correct settings are applied for each environment, enhancing the project’s adaptability and ease of deployment.
Optimizing Maven Builds
Efficiency in build processes is important for developer productivity. Maven offers several options to optimize builds:
Parallel Builds: Use the -T or –threads option to specify the number of threads for parallel builds, speeding up the build process by taking advantage of multi-core processors.
mvn install -T 4
Offline Mode: The -o or –offline switch forces Maven to work offline, using only the dependencies already downloaded to the local repository. This can save time and bandwidth.
mvn package -o
Incremental Builds: Maven does not have a built-in incremental build feature, but you can use the -pl, -am, and -amd flags to build only a specific project and its dependencies, making the build faster by avoiding unnecessary work.
mvn install -pl my-module -am
These techniques help developers reduce build times and improve efficiency, making Maven an even more powerful tool in the development process.
Troubleshooting Common Maven Issues
Even with a robust tool like Maven, developers may encounter issues. Here are strategies to troubleshoot common Maven problems:
- Dependency Conflicts: Conflicts occur when different modules of your project require different versions of the same dependency. Use mvn dependency:tree to identify and resolve conflicts by finding the appropriate version that satisfies all modules.
- Plugin Errors: Plugin errors often arise from misconfigurations or version incompatibilities. Ensure you are using the correct plugin version compatible with your project’s Maven version. Check the plugin’s documentation for required configurations.
- Build Failures: Build failures can stem from a variety of issues, including code compilation errors, test failures, or environmental problems. Review the build error messages carefully; they often provide specific information about what went wrong and how to fix it. Running Maven in debug mode (mvn -X) can provide additional insights.
Addressing these issues typically involves inspecting the output logs, understanding the problem, and applying the appropriate fix. Whether it is adjusting dependencies, updating plugin configurations, or fixing code errors, a methodical approach to troubleshooting can resolve most Maven-related issues.
Conclusion
This Maven Options Command Cheat Sheet serves as a guide to harnessing Maven’s capabilities effectively, from basic commands to advanced configurations and troubleshooting common issues. Maven streamlines the build process, enhances project management, and facilitates dependency management, making it an indispensable tool for Java developers. By mastering the commands and strategies outlined in this cheat sheet, developers can optimize their workflow, ensuring efficient and successful project builds. Embrace these Maven practices to elevate your development process, encouraging a culture of productivity and excellence in your Java projects.