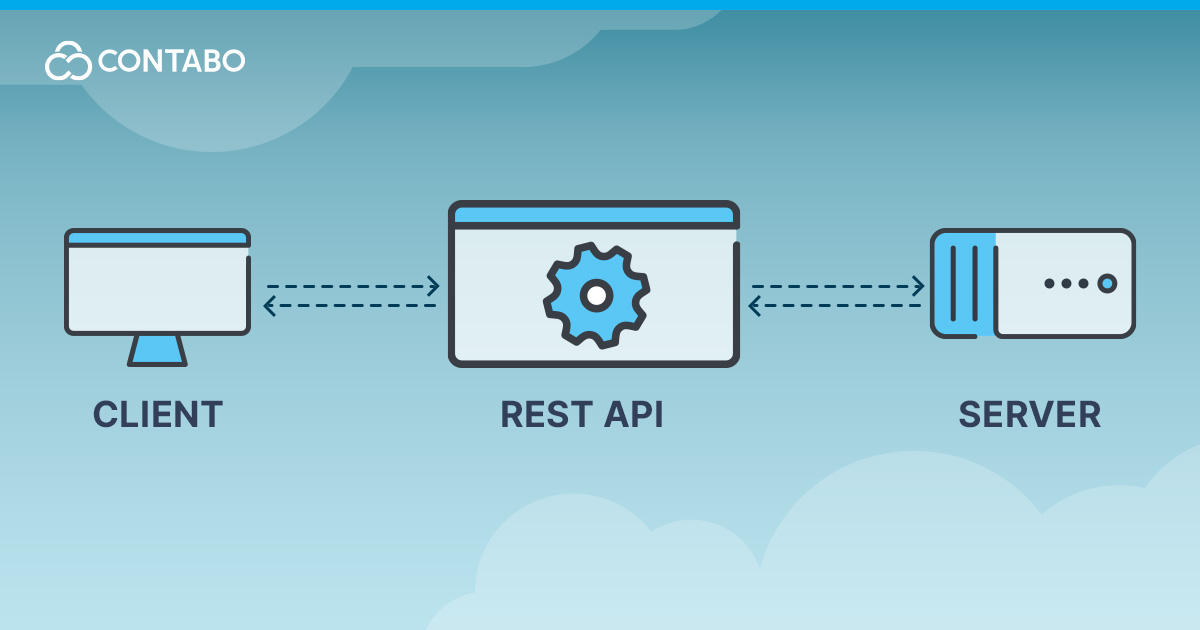
A REST API (Representational State Transfer) is pivotal in modern software development, enabling seamless interaction between distributed systems. These interfaces facilitate the exchange of data and functionality through a set of stateless operations. As a result, they are essential for developers and Linux system administrators aiming to build or maintain scalable, efficient web services.
This guide introduces the basics of REST APIs, from their underlying principles to practical implementations. As we explore how these APIs can streamline server-client communication, our focus is on empowering you with the knowledge to apply REST principles in your own projects. This will enhance interoperability and performance across diverse IT environments. By the end of this section, you’ll have a clear understanding of why REST APIs are favored in application development and how they can be harnessed effectively.
What is REST API
REST, an acronym for Representational State Transfer, serves as the foundation for a widely adopted architectural style in web services and application programming interfaces (APIs). RESTful services leverage the existing protocols of the web, primarily HTTP, to manage interactions between client and server applications. The primary appeal of REST lies in its straightforward approach. This approach not only promotes scalability and performance but also facilitates ease of integration.
Principles of REST Architecture
REST is grounded in six fundamental principles that guide its architecture and operations:
- Client-Server Separation: The client and the server operate independently, allowing each to be developed, replaced, and scaled without affecting the other. This separation simplifies components on both ends, promoting clear interfaces and better maintainability.
- Statelessness: Each request from the client to the server must contain all the information the server needs to understand and respond to the request. The server does not store any state about the client session on the server side, which enhances reliability and scalability.
- Cacheability: Responses must define themselves as cacheable or non-cacheable. If a response is cacheable, the client cache is given the rights to reuse that response data for later, equivalent requests. Caching can eliminate some client-server interactions, significantly improving efficiency and speed.
- Uniform Interface: To simplify the architecture and decouple the services from their clients, REST insists on a uniform interface that standardizes the interactions between clients and servers. This principle encompasses four key constraints:
- Resource-Based: Individual resources are identified in requests using URIs as resource identifiers.
- Manipulation of Resources Through Representations: Clients interact with resources through their representations, such as JSON or XML, which carry enough information to modify or delete the resource on the server.
- Self-descriptive Messages: Each message includes enough information to describe how to process the message.
- Hypermedia as the Engine of Application State (HATEOAS): Clients dynamically discover available actions and resources via hypermedia provided by the server.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server, or to an intermediary along the way. Intermediate servers can improve system scalability by enabling load balancing and providing shared caches.
- Code on Demand (optional): Servers can temporarily extend or customize the functionality of a client by transferring executable code. This is the only optional constraint of REST.
Key Components of a REST API
A REST API is composed of several key elements that define its structure and functionality. Understanding these components is essential for developers and administrators who design, develop, or integrate APIs into their systems.
Resources
At the core of any REST API are the resources, which represent any kind of object, data, or service accessible by the client. Each resource is uniquely identified by a URI (Uniform Resource Identifier) and is manipulated through representations. For instance, in an API for a social media platform, resources might include individual user profiles, photos, or comments.
Requests and Responses
Interactions with resources are conducted using standard HTTP methods, which are fundamental operations of REST APIs:
- GET: Retrieves data from the server. Using GET should only fetch data and not change any state of the application.
- POST: Sends data to the server to create a new resource. It is used when the client submits information to be processed to the server.
- PUT: Updates existing resources. If the resource does not exist, it can optionally be created.
- DELETE: Removes resources from the server.
Each of these requests will elicit a response from the server. This response typically includes a status code indicating success or failure of the request, and, in cases like a GET request, it includes the data of the requested resource.
Methods of REST API
In addition to the basic methods described above, REST APIs also support:
- PATCH: Applies partial modifications to a resource. It is useful for updates that affect only certain attributes of a resource rather than replacing the entire resource, as PUT might.
The choice between PUT and PATCH can be significant: PUT replaces an entire resource whereas PATCH modifies it, making PATCH more bandwidth-efficient for minor changes.
How REST APIs Work
REST APIs operate on a straightforward yet powerful client-server architecture, which is fundamental to understanding their functionality and widespread adoption in software development. This architecture enables REST APIs to facilitate seamless interactions between clients and servers. Moreover, by maintaining a stateless communication protocol, they enhance performance and scalability.
REST Client-Server Architecture
In the RESTful model, the client and server are distinct components that interact through HTTP requests and responses. The client initiates requests to perform various operations (like retrieving, creating, updating, or deleting data), and the server processes these requests and returns appropriate responses. This separation of concerns allows for greater flexibility and scalability, as the client and server evolve independently without direct dependencies on one another.
Stateless Interactions
One of the core principles of REST is statelessness. This means that each request from a client to a server must contain all the information necessary for the server to understand and process the request. The server does not store any session information about the client; instead, each interaction is processed in isolation. This approach simplifies the server design and improves scalability because the server does not need to maintain, manage, or communicate session state.
Resource Representations
When a client makes a request to a server, it seeks to interact with a resource. In REST APIs, resources are not just data but also the representations of that data. For example, a client can request a resource in formats like JSON, XML, or HTML. The server responds with the resource in the requested format, along with metadata in the HTTP headers to help the client process the response correctly. This flexibility enables various types of clients (web browsers, mobile apps, other servers) to interact with the API in the way that best suits their needs.
Implementing REST APIs
Implementing a REST API involves setting up a server that can handle HTTP requests and send appropriate responses based on the operations requested by clients. This section first outlines the basic steps and then delves into the key considerations in creating a RESTful server.
How to Set Up a Basic REST Server
The first step in implementing a REST API is to set up a server that can handle HTTP requests. This usually involves choosing a server-side language and framework that supports REST architecture, such as Node.js with Express, Python with Flask, or Java with Spring Boot. Here is a simple example using Python and Flask:
from flask import Flask, jsonify, request
app = Flask(__name__)
# A simple in-memory structure to store data
data = {
'items': [{'id': 1, 'name': 'Item One'}]
}
# Endpoint to retrieve items
@app.route('/items', methods=['GET'])
def get_items():
return jsonify(data)
# Endpoint to add a new item
@app.route('/items', methods=['POST'])
def add_item():
item = {'id': len(data['items']) + 1, 'name': request.json['name']}
data['items'].append(item)
return jsonify(item), 201
# Endpoint to update an item
@app.route('/items/<int:item_id>', methods=['PUT'])
def update_item(item_id):
item = next((item for item in data['items'] if item['id'] == item_id), None)
if not item:
return jsonify({'message': 'Item not found'}), 404
item['name'] = request.json['name']
return jsonify(item)
# Endpoint to delete an item
@app.route('/items/<int:item_id>', methods=['DELETE'])
def delete_item(item_id):
item = next((item for item in data['items'] if item['id'] == item_id), None)
if not item:
return jsonify({'message': 'Item not found'}), 404
data['items'].remove(item)
return jsonify({'message': 'Item deleted'})
if __name__ == '__main__':
app.run(debug=True)
This simple server setup showcases how to handle GET, POST, PUT, and DELETE requests, which are the backbone of RESTful services. Additionally, it demonstrates the stateless nature of REST, where each request must independently provide all necessary information.
Handling Requests and Responses
When developing a REST API, handling requests correctly is important. The server must interpret what the client wants (whether it is retrieving, creating, updating, or deleting data), and the responses must be clear and informative. For instance, when a GET request succeeds, the server returns a 200 OK status with the data. If something goes wrong, use appropriate HTTP status codes. Use 404 Not Found for an invalid resource request. Use 500 Internal Server Error for server issues.
Properly managing these interactions is key to building a robust and user-friendly API. It not only ensures the reliability of the API but also enhances its usability by providing clear and consistent feedback to the client about the outcome of their requests.
By following these guidelines, developers can create effective RESTful APIs. These APIs are maintainable and scalable. They are ideal for a wide range of applications in diverse environments.
Best Practices for REST API Development
Developing REST APIs involves more than just handling requests and responses. Following best practices can significantly enhance the security, efficiency, and maintainability of your APIs. Here, we outline essential strategies for robust REST API development.
Security Considerations
Security is paramount in API development to protect data and ensure only authorized access:
- Authentication and Authorization: Implement robust authentication mechanisms to verify users before they can interact with your API. OAuth and JWT (JSON Web Tokens) are popular choices for managing secure access.
- HTTPS: Use HTTPS to encrypt data transmitted between the client and the server, safeguarding against interception and tampering.
- Input Validation: Always validate inputs to avoid SQL injection, cross-site scripting (XSS), and other malicious attacks. Consequently, proper validation ensures that inputs conform to expected formats.
- Rate Limiting: Prevent abuse and DoS (Denial of Service) attacks by limiting how often a user or IP can make requests within a certain period.
Efficient Use of Resources and Caching
Optimizing the performance of your API is important for providing a good user experience and reducing server load:
- Data Pagination: For endpoints that could return large volumes of data, use pagination to limit the response size. This not only speeds up response times but also reduces memory usage and network congestion.
- Caching: Implement caching strategies to store responses where possible. Caching decreases the number of times resources need fetching from the server, reducing latency and server load. Use HTTP headers like ETag and Last-Modified to manage cache validity.
- Resource Minimization: Only send necessary data in responses to minimize bandwidth. Use techniques like filtering, sorting, and field selection to allow clients to specify exactly what they need.
Conclusion
In this guide, we explored the core principles of REST APIs. Firstly, we highlighted their role in building scalable and maintainable web services. Furthermore, by understanding and applying REST concepts, developers and system administrators can significantly enhance the performance, security, and efficiency of their applications.
As you explore REST API development, remember a few key points. Firstly, adherence to best practices is important. Additionally, a solid grasp of stateless communication and HTTP protocols is crucial for success. This knowledge will empower you to craft robust solutions that effectively manage data and services across diverse environments. Finally, embrace the potential of REST APIs to elevate your web development projects and streamline your integration processes.