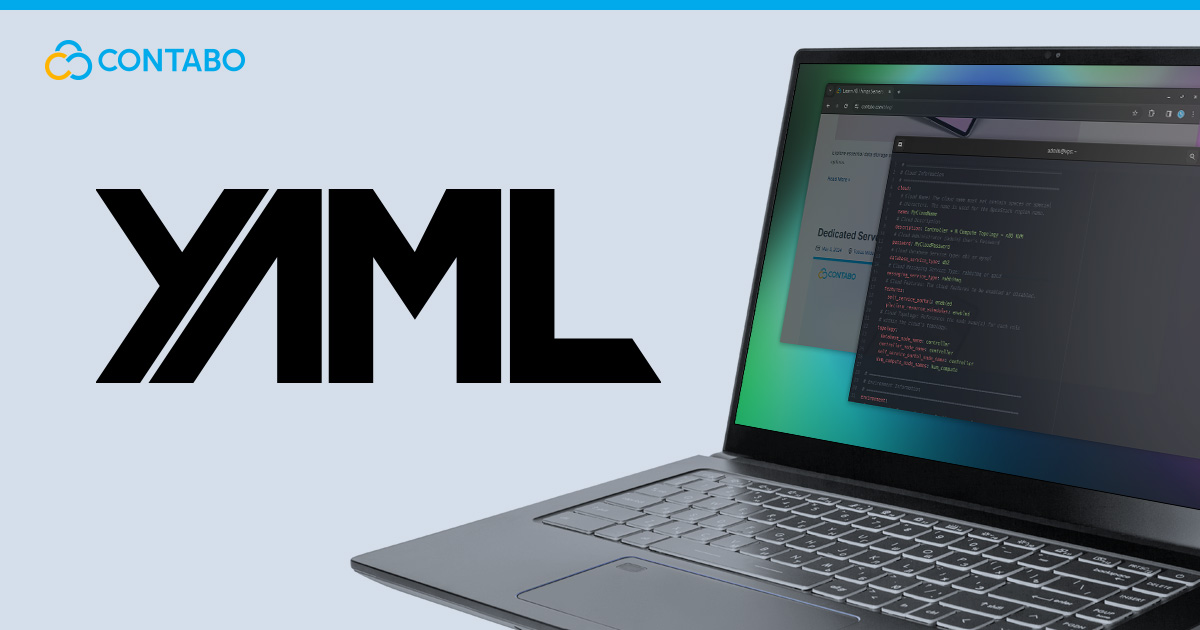
YAML, which stands for “YAML Ain’t Markup Language,” is a human-readable data serialization standard that is commonly used for configuration files and data exchange between languages with different data structures. It is designed to be simple and easy to understand, making it a popular choice for developers and system administrators.
What is YAML?
YAML is a data serialization language that emphasizes human readability. Unlike XML or JSON, which can become complex and hard to read, YAML uses a minimalist syntax that is both intuitive and expressive. This makes it an excellent choice for configuration files and data exchange formats where ease of use and clarity are top priorities.
Why Use YAML?
There are several reasons why YAML is widely used:
- Readability: YAML’s syntax is clean and easy to read, making it simple to write and understand. This readability reduces the likelihood of errors and makes maintenance easier.
- Flexibility: YAML supports complex data structures, including nested data, making it versatile for various applications.
- Compatibility: YAML is compatible with many programming languages, including Python, Ruby, and JavaScript. This makes it easy to integrate into existing projects.
- Minimal Syntax: YAML uses indentation and simple syntax rules, which reduces the need for punctuation and other special characters, making files less cluttered and more maintainable.
In summary, YAML’s focus on readability, flexibility, and minimal syntax makes it an ideal choice for developers and system administrators looking for a simple yet powerful configuration and data serialization format.
Basic Syntax of YAML
Understanding the basic syntax of YAML is essential for creating and managing YAML files effectively. YAML’s syntax is designed to be easy to read and write, focusing on simplicity and clarity.
Structure and Indentation
YAML relies heavily on indentation to represent the structure and hierarchy of the data. Unlike other formats that use braces or brackets, YAML uses spaces to denote levels of nesting. Here are some key points to remember:
- Indentation: Use spaces, not tabs, for indentation. The number of spaces used must be consistent throughout the document.
- Nested Structures: Indentation indicates nested structures, like how Python handles them.
Example:
person:
name: John Doe
age: 30
address:
street: 123 Main St
city: Anytown
In this example, name, age, and address are keys under the person key, and street and city are keys under the address key.
Keys and Values
In YAML, data is represented as key-value pairs. Keys are always strings, and values can be various data types, including strings, numbers, lists, and dictionaries.
Example:
key: value
Keys and values are separated by a colon and a space. Keys must be unique within the same level of hierarchy.
Comments in YAML
In YAML comments begin with the # character and extend to the end of the line. Comments are useful for adding notes or explanations within the YAML file.
Example:
# This is a comment
key: value # This is an inline comment
Scalars (Strings, Numbers, Booleans)
Scalars are single, indivisible values such as strings, numbers, and booleans. YAML supports several ways to define scalar values:
- Strings: Strings can be enclosed in quotes or written plainly.
plain_string: Hello, World!
quoted_string: "Hello, World!"
- Numbers: Numbers can be written plainly.
integer: 123
float: 123.45
- Booleans: Boolean values can be true or false.
boolean: true
By understanding these basic syntax rules, you can start creating and managing YAML files effectively. The simplicity and readability of YAML make it a powerful tool for configuration and data serialization.
YAML Data Types
YAML supports a variety of data types, which allows it to handle complex data structures. Understanding these data types is important for effectively using YAML in your projects.
Scalars
Scalars are the most basic data types in YAML and include strings, numbers, and booleans. They represent single, indivisible values.
- Strings: Strings can be written with or without quotes. Double quotes allow for special characters, while single quotes are more literal.
plain_string: Hello, World!
quoted_string: "Hello, World!"
single_quoted_string: 'Hello, World!'
- Numbers: Numbers are written without quotes and can be integers or floating-point values.
integer: 123
float: 123.45
- Booleans: Boolean values are written as true or false.
boolean_true: true
boolean_false: false
Sequences (Lists)
Sequences in YAML are collections of items. They are defined using a dash followed by a space and each item on a new line with the same level of indentation.
Example:
fruits:
- Apple
- Orange
- Banana
In this example, fruits is a key with a list of string values.
Mappings (Dictionaries)
Mappings, also known as dictionaries, are collections of key-value pairs. They are defined using indentation to represent nested structures.
Example:
person:
name: Jane Doe
age: 25
contact:
email: [email protected]
phone: 123-456-7890
Here, person is a key with nested key-value pairs, and contact is a nested dictionary within person.
Null Values
YAML can also represent null values using null or ~.
Example:
unknown: null
missing: ~
Understanding these data types helps you leverage the full power of YAML’s data representation capabilities. Whether you are defining simple key-value pairs or complex nested structures, YAML’s flexible and readable syntax makes it an ideal choice for many applications.
Creating and Using YAML Files
Creating and using YAML files involves writing the YAML content in a text editor and then reading or parsing the file in a programming language, such as Python. This section will guide you through the basics of writing and reading YAML files.
Writing a YAML File
To create a YAML file, follow these steps:
- Choose a Text Editor: Use a text editor that supports YAML syntax highlighting for better readability and fewer errors. Examples include VSCode, Sublime Text, and Notepad++.
- Create the YAML Content: Write the YAML content following the syntax rules discussed earlier.
Example:
person:
name: John Doe
age: 30
contact:
email: [email protected]
phone: 123-456-7890
- Save the File: Save the file with a .yaml or .yml extension. For instance, example.yaml.
Reading a YAML File in Python
Python’s PyYAML library makes it easy to read and write YAML files. Here is a simple example of how to read a YAML file in Python.
- Install PyYAML: First, you need to install the PyYAML library if you have not already.
pip install pyyaml
- Read the YAML File: Use the following Python code to read the YAML file.
Example:
import yaml
# Open the YAML file
with open('example.yaml', 'r') as file:
# Load the YAML content
data = yaml.safe_load(file)
# Print the loaded data
print(data)
In this example, the yaml.safe_load() function reads the YAML content and converts it into a Python dictionary.
Writing a YAML File in Python
You can also write data to a YAML file using the PyYAML library. Here is how:
Example:
import yaml
# Define data to be written to YAML
data = {
'person': {
'name': 'John Doe',
'age': 30,
'contact': {
'email': '[email protected]',
'phone': '123-456-7890'
}
}
}
# Write the data to a YAML file
with open('output.yaml', 'w') as file:
yaml.dump(data, file)
In this example, the yaml.dump() function writes the Python dictionary to a YAML file.
YAML vs JSON
YAML and JSON are both widely used formats for data serialization and configuration files. While they share some similarities, they also have distinct differences. Below is a comparison table highlighting the key differences between YAML and JSON.
Feature | YAML | JSON |
Readability | Highly readable and human-friendly | Less readable due to extensive use of braces and brackets |
Syntax | Uses indentation and spaces, avoiding special characters like commas and braces | Uses braces {}, brackets [], and commas , for structure |
Data Types | Supports complex data types, including references and multi-line strings | Supports basic data types such as strings, numbers, arrays, and objects |
Use Cases | Ideal for configuration files and data serialization in human-facing applications | Commonly used for APIs and data interchange between web services |
File Size | Generally larger due to additional formatting for readability | More compact, making it efficient for data transfer |
Comments | Supports comments with # | Does not natively support comments |
Learning Curve | Easy to learn for those familiar with Python-like indentation | Simple syntax but less intuitive due to strict formatting requirements |
Flexibility | Highly flexible with support for various data structures | Less flexible but highly standardized |
When to Use YAML vs. JSON
- Use YAML:
- When you need a readable and writable configuration file format.
- For complex data structures that benefit from YAML’s flexible syntax.
- When working with human-facing applications where clarity is essential.
- Use JSON:
- For web APIs and data interchange between client and server.
- When working with applications that require lightweight and straightforward data serialization.
- In scenarios where strict syntax and easy parsing by machines are priorities.
Choosing between YAML and JSON depends on the specific requirements of your project. YAML is favored for its readability and flexibility, making it suitable for configuration files and human-readable data. JSON, on the other hand, is widely used in web development for its simplicity and efficiency in data interchange.
Conclusion
YAML is a powerful and flexible data serialization language that excels in readability and simplicity. Its minimal syntax and use of indentation make it an ideal choice for configuration files, data serialization, and various other applications where human readability is essential.
Key points to remember about YAML
- Readability: YAML’s syntax is clean and easy to understand, which reduces errors and simplifies maintenance.
- Flexibility: YAML supports complex data structures, including nested objects, lists, and dictionaries.
- Compatibility: YAML is widely supported across many programming languages, making it easy to integrate into existing projects.
- Use Cases: YAML is particularly well-suited for configuration files, document storage, and data serialization.
Whether you are a Linux system administrator, programmer, or developer, mastering YAML can significantly enhance your ability to manage and configure applications efficiently. By leveraging YAML’s strengths, you can create more maintainable and readable configurations, ensuring smoother workflows and better collaboration across your projects.