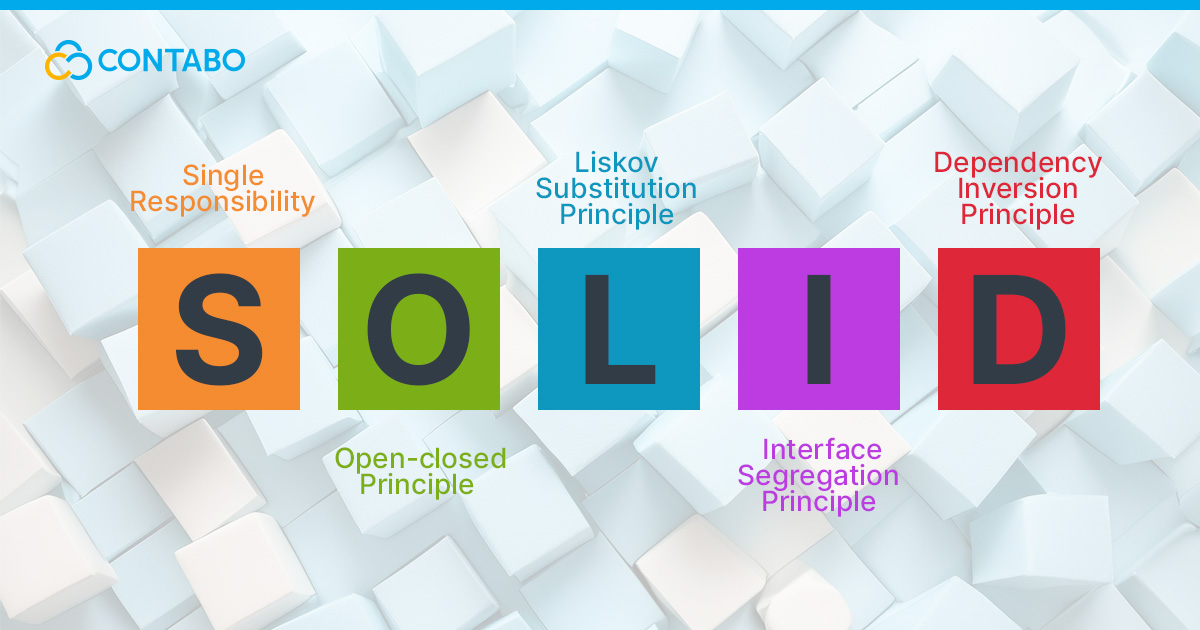
SOLID principles are a set of guidelines that help software developers write maintainable, scalable, and flexible code. The acronym SOLID stands for five fundamental principles of object-oriented programming: Single Responsibility Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle. This article covers all five SOLID principles in detail, with each principle explained in its own section.
These principles were introduced by Robert C. Martin (also known as Uncle Bob) in the early 2000s and have since become widely adopted in the software development community. Martin, a pioneer in software craftsmanship and agile methodologies, has authored influential books like “Clean Code.” By following SOLID principles, developers can create code that is easier to understand, modify, and extend, leading to more robust and maintainable software systems.
Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) is one of the fundamental principles of object-oriented programming and SOLID design. It states that a class should have only one reason to change, meaning it should have a single responsibility or job.
Definition and Explanation
According to the SRP, a class should be designed to handle a specific task or responsibility. If a class has more than one responsibility, it becomes harder to understand, modify, and maintain over time. When a class needs to be changed, the change should be specific to only one of its responsibilities, not affecting the other responsibilities it handles.
Benefits of Following SRP
Adhering to the Single Responsibility Principle offers several benefits:
- Improved Code Organization: By separating concerns into different classes, the codebase becomes more organized and easier to navigate.
- Better Maintainability: When a class has a single responsibility, it is easier to understand its purpose and make changes without unintended side effects.
- Increased Reusability: Classes with a single responsibility are more likely to be reusable in different parts of the application or even in other projects.
- Easier Testing: Classes with a single responsibility are typically smaller and more focused, making them easier to test in isolation.
Open/Closed Principle (OCP)
The Open/Closed Principle (OCP) is a core principle of the SOLID principles in object-oriented programming. Introduced by Bertrand Meyer, a renowned software engineer and author, the OCP states that software entities (such as classes, modules, and functions) should be open for extension but closed for modification.
Definition and Explanation
The Open/Closed Principle means that developers should be able to add new functionality to a class without changing its existing implementation. This can be achieved through techniques such as abstraction, inheritance, and polymorphism. By adhering to the OCP, developers can create software systems that are more maintainable, scalable, and flexible.
Benefits of Following OCP
Adhering to the Open/Closed Principle offers several benefits:
- Reduced Risk of Bugs: By not modifying existing code, the risk of introducing new bugs or breaking existing functionality is minimized.
- Improved Maintainability: Code that follows the OCP is easier to maintain and extend, as new features can be added without altering the existing codebase.
- Enhanced Flexibility: The use of abstractions and polymorphism allows for more flexible and adaptable designs, making it easier to accommodate changing requirements.
Best Practices for Implementing OCP
To effectively implement the Open/Closed Principle in your codebase, consider the following best practices:
- Use Abstraction: Define abstract classes or interfaces to represent common behaviors and allow for different implementations.
- Leverage Polymorphism: Use polymorphism to enable different implementations to be used interchangeably.
- Avoid Tight Coupling: Design your classes to be loosely coupled, making it easier to extend functionality without modifying existing code.
- Follow Design Patterns: Utilize design patterns such as Strategy, Template Method, and Visitor to implement the Open/Closed Principle effectively.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) is another important principle in the SOLID set of principles for object-oriented programming. It was introduced by Barbara Liskov, a pioneering computer scientist and Turing Award winner, and is named after her.
Definition and Explanation of LSP
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. In other words, if S is a subtype of T, then objects of type T may be replaced with objects of type S (i.e., objects of type S may be substituted for objects of type T) without altering any of the desirable properties of the program. This principle is based on the concept of subtyping, which is a way to establish a hierarchical relationship between types. A subtype is a type that inherits from another type (the supertype) and can be used in place of the supertype.
Benefits of Following LSP
Adhering to the Liskov Substitution Principle offers several benefits:
- Improved Code Reusability: By ensuring that subtypes can be substituted for their base types, code that uses the base type can also work with any of its subtypes, promoting code reuse.
- Enhanced Maintainability: Code that follows LSP is easier to maintain because it reduces the risk of introducing bugs when modifying or extending the codebase.
- Better Testability: LSP makes it easier to write unit tests for classes and their subtypes, as the tests can be written against the base type and should work for all subtypes.
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) is one of the SOLID design principles that help in creating modular and maintainable software systems. It states that “Clients should not be forced to depend on interfaces they do not use.” In simpler terms, ISP suggests we break down large interfaces into smaller, more specific ones. This way, clients (classes or modules) only need to depend on the methods they require. This principle promotes loose coupling and high cohesion, making the code more modular, reusable, and easier to maintain.
Benefits of Using Interface Segregation Principle
- Modular and Reusable Code: By breaking down large interfaces into smaller, more specific ones, the code becomes more modular and reusable. Classes or modules can implement only the interfaces they need, reducing unnecessary dependencies and making it easier to reuse code across different parts of the system.
- Reduced Code Complexity: When classes or modules depend only on the interfaces they need, the code becomes less complex and easier to understand. This is because developers do not have to deal with unnecessary methods or dependencies. These are not relevant to their specific use case.
- Improved Maintainability: With smaller and more focused interfaces, it becomes easier to maintain the code. Changes to one interface are less likely to affect other parts of the system, reducing the risk of introducing bugs or breaking existing functionality.
- Better Testability: Smaller and more focused interfaces make it easier to write unit tests for individual components. This is because the tests can focus on specific behaviors without being affected by irrelevant methods or dependencies.
- Increased Flexibility: By adhering to the ISP, the system becomes more flexible and easier to extend or modify. New features or requirements can be added by creating new interfaces or modifying existing ones without affecting the entire system.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) is the final principle in the SOLID design principles. It was introduced by Robert C. Martin, a key figure in software engineering, and is important for creating loosely coupled and maintainable software systems.
Definition and Explanation
The Dependency Inversion Principle states that:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In simpler terms, DIP suggests that the high-level policy-setting modules should not depend on the low-level, implementation-specific modules. Instead, both should depend on interfaces or abstract classes. This inversion of dependency helps in achieving a more flexible and resilient architecture.
Benefits of Following DIP
Adhering to the Dependency Inversion Principle offers several benefits:
- Loose Coupling: By depending on abstractions rather than concrete implementations, the code becomes less tightly coupled, making it easier to change one part of the system without affecting others.
- Improved Maintainability: Changes in low-level modules do not impact high-level modules, making the system easier to maintain and extend.
- Enhanced Testability: High-level modules can be tested using mock implementations of the low-level modules, making testing faster and more reliable.
- Increased Reusability: High-level modules can be reused in different contexts without needing to change the low-level modules they depend on.
Best Practices for Implementing DIP
- Use Interfaces and Abstract Classes: Define interfaces or abstract classes to represent the dependencies between high-level and low-level modules.
- Dependency Injection: Use dependency injection to provide the dependencies to a class, rather than having the class create its own dependencies.
- Avoid Tight Coupling: Design your classes to be loosely coupled, making it easier to extend functionality without modifying existing code.
- Follow Other SOLID Principles: Adhering to the Open/Closed Principle and the Liskov Substitution Principle can help in implementing the Dependency Inversion Principle effectively.
Applying SOLID in Practice
While the SOLID principles provide a solid foundation for writing maintainable and extensible code, applying them in practice can be challenging, especially in large and complex codebases. In this chapter, we will discuss some of the challenges developers may face when adopting SOLID principles. We will also provide best practices and guidelines to help overcome them.
Challenges in Adopting SOLID Principles
- Legacy Code: Refactoring legacy code to adhere to SOLID principles can be a daunting task, as it may require significant changes to the existing codebase.
- Team Buy-in: Ensuring that all team members understand and consistently apply SOLID principles can be challenging, especially in larger teams or organizations.
- Balancing Trade-offs: In some cases, adhering strictly to SOLID principles may lead to increased complexity or performance overhead, requiring developers to balance trade-offs between maintainability and other concerns.
- Overengineering: There is a risk of overengineering the codebase by applying SOLID principles too rigidly or prematurely, leading to unnecessary complexity and reduced productivity.
SOLID Best Practices and Guidelines
- Incremental Refactoring: Instead of attempting a complete overhaul, gradually refactor the codebase to adhere to SOLID principles, focusing on areas with high technical debt or frequent changes.
- Code Reviews and Pair Programming: Encourage code reviews and pair programming to ensure consistent application of SOLID principles and to share knowledge within the team.
- Automated Testing: Implement comprehensive automated testing to ensure that refactoring efforts do not introduce regressions and to facilitate future changes.
- Continuous Learning: Encourage continuous learning and knowledge sharing within the team. This includes studying real-world examples and best practices for applying SOLID principles.
- Balance Principles with Pragmatism: While SOLID principles are valuable guidelines, developers should also consider the specific context and requirements of their project. They should balance maintainability with other concerns such as performance and simplicity.
- Embrace Design Patterns: Leverage proven design patterns, such as the Strategy, Observer, and Factory patterns. These patterns often align with and facilitate the application of SOLID principles.
- Prioritize Readability and Simplicity: While adhering to SOLID principles, prioritize code readability and simplicity, avoiding unnecessary complexity or over-abstraction.
Impact on Code Maintainability and Extensibility
By consistently applying SOLID principles, developers can create code that is more maintainable, extensible, and resilient to change. Adhering to these principles promotes loose coupling, high cohesion, and separation of concerns. This makes it easier to understand, modify, and extend the codebase over time.
Well-designed, SOLID-compliant code is also more testable. It enables developers to create modular and isolated components that can be tested independently. This, in turn, increases confidence in the codebase and reduces the risk of introducing regressions during future development.
While the initial effort required to adopt SOLID principles may be higher, the long-term benefits make it worthwhile. These benefits include reduced technical debt, increased productivity, and improved code quality for any software development project.
SOLID Cheat Sheet
Principle | Description |
Single Responsibility Principle (SRP) | A class should have only one reason to change, or in other words, it should have a single responsibility. |
Open/Closed Principle (OCP) | Software entities should be open for extension but closed for modification. |
Liskov Substitution Principle (LSP) | Subtypes must be substitutable for their base types without altering the correctness of the program. |
Interface Segregation Principle (ISP) | Clients should not be forced to depend on interfaces they do not use. |
Dependency Inversion Principle (DIP) | High-level modules should not depend on low-level modules; both should depend on abstractions. Abstractions should not depend on details, but details should depend on abstractions. |
Conclusion
The SOLID principles are a set of fundamental guidelines. They can help developers create more maintainable, extensible, and robust object-oriented software systems. By adhering to these principles, code becomes easier to understand, modify, and scale over time.
Although originally conceived for object-oriented design, developers can apply the core ideas behind SOLID more broadly. They are relevant to other programming paradigms as well. The principles encourage modular design, separation of concerns, and loose coupling between components. These are beneficial practices in any software development approach.
It is important to note that developers should apply SOLID principles judiciously. Consideration for the project’s specific context and requirements is essential. Blindly following the principles without understanding their intent can lead to over-engineering and unnecessary complexity.
The true value of SOLID lies in its ability to foster code that is flexible, maintainable, and resilient to change. When developers embrace these principles, they can create software systems better equipped to adapt to evolving requirements and technologies. This leads to increased productivity and reduced technical debt.