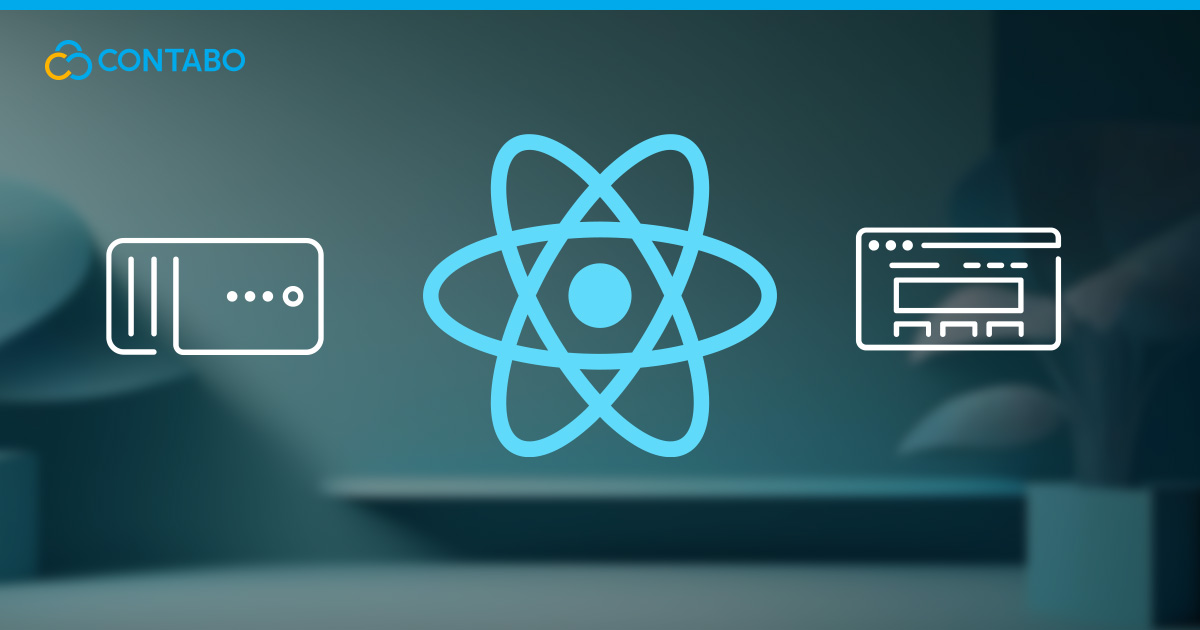
Server-side rendering (SSR) is an important technique in modern web development, particularly for React applications. SSR refers to the process of rendering a JavaScript-based web application on the server rather than in the client’s browser. This approach offers several advantages for both developers and end-users.
What is SSR?
In the context of React, SSR involves generating the initial HTML content of a web page on the server before sending it to the client. This means that when a user requests a page, the server executes the React code, creates the HTML structure, and sends the fully rendered page to the browser. The client then takes over and handles subsequent interactions and updates.
Benefits of SSR
- Improved Initial Load Time: SSR can significantly reduce the time it takes for users to see and interact with content, especially on slower devices or networks.
- Enhanced SEO: Search engines can more easily crawl and index server-rendered content, potentially improving search rankings.
- Better Performance on Low-powered Devices: By offloading the initial rendering to the server, SSR reduces the computational burden on the client device.
- Improved User Experience: Users see content faster, leading to a more responsive feel and potentially lower bounce rates.
SSR in React provides a powerful way to create fast, SEO-friendly, and user-centric web applications. As we delve deeper into this guide, we will explore how SSR works, how to implement it, and the key considerations for using this approach in your React projects.
How Server-side Rendering Works
Server-side rendering (SSR) is the process of generating web page content on the server before transmitting it to the client’s browser. This method provides a faster initial loading experience, as the server sends fully rendered HTML to the client, which can immediately display the content rather than waiting for JavaScript to execute.
The SSR Process
When a user requests a web page, the server handles the request by processing the necessary data and rendering it into HTML output. This HTML is then sent to the client’s browser, where it can be displayed immediately. Here is a step-by-step breakdown of the SSR process:
- User Request: A user enters a URL in the browser’s address bar.
- Server Processing: The server receives the request, processes the data, and renders the HTML.
- HTML Delivery: The server sends the fully rendered HTML back to the client’s browser.
- Client Display: The browser displays the HTML content immediately.
- Client-side Hydration: JavaScript code is executed to make the page interactive, a process known as hydration.
Advantages of SSR
- Faster Load Times: Since the server delivers pre-rendered HTML, users can see content more quickly, improving the perceived performance of the application.
- SEO Benefits: Search engine crawlers can effectively index web content without executing any client-side scripts, leading to better SEO performance.
- Consistent Experience: Ensures a consistent user experience across different browsers, as the server handles the rendering.
Comparison with Client-side Rendering (CSR)
While SSR is ideal for scenarios where speed and SEO are important, client-side rendering (CSR) allows for a more dynamic and interactive user experience once the application is loaded. CSR renders content in the browser using JavaScript after the initial HTML page has loaded, meaning that some content may take longer to become visible to users compared to server-rendered pages. In conclusion, SSR serves as a great approach for applications where initial loading speed and SEO are important priorities, providing a faster, more consistent experience for users.
Setting Up a Basic SSR React Application
Setting up a basic server-side rendering (SSR) React application involves several steps, including installing necessary tools, creating a simple React app, and configuring the server to render the app. Here is a step-by-step guide to get you started.
Required Tools and Dependencies
To set up SSR in React, you need the following tools and dependencies:
- Node.js: A JavaScript runtime for server-side code.
- Express: A web framework for Node.js to handle server requests.
- Babel: A JavaScript compiler to transform JSX and ES6+ code into browser-compatible JavaScript.
Creating a Simple SSR React App
- Initialize the Project:
npx create-react-app ssr-example
cd ssr-example
npm install express react react-dom @babel/core @babel/preset-env @babel/preset-react babel-loader
- Set Up Babel Configuration: Create a .babelrc file in the project root:
{
"presets": ["@babel/preset-env", "@babel/preset-react"]
}
- Create the Server File: Create a server.js file in the project root:
const express = require('express');
const React = require('react');
const ReactDOMServer = require('react-dom/server');
const App = require('./src/App').default;
const app = express();
app.use(express.static('build'));
app.get('*', (req, res) => {
const appString = ReactDOMServer.renderToString(<App />);
const html = `
<!DOCTYPE html>
<html>
<head>
<title>SSR React App</title>
</head>
<body>
<div id="root">${appString}</div>
<script src="/static/js/bundle.js"></script>
</body>
</html>
`;
res.send(html);
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
- Modify the React App: Ensure your App.js is exportable:
import React from 'react';
const App = () => {
return (
<div>
<h1>Hello, SSR!</h1>
</div>
);
};
export default App;
- Build and Run the Application:
npm run build
node server.js
Visit http://localhost:3000 to see your server-rendered React application in action.
Challenges and Considerations in SSR
Implementing server-side rendering (SSR) in React applications comes with its own set of challenges and considerations. Understanding these can help you make informed decisions and optimize your application effectively.
Performance Implications
While SSR can improve initial load times, it can also introduce performance bottlenecks on the server. Rendering React components on the server requires computational resources, which can become a limiting factor under high traffic. To mitigate this, consider:
- Caching: Cache rendered pages to reduce server load.
- Load Balancing: Distribute requests across multiple servers to handle high traffic.
SEO Benefits
One of the primary advantages of SSR is improved search engine optimization (SEO). Since the content is rendered on the server, search engines can index the content more effectively. This can lead to better visibility in search results. However, ensure that:
- Meta Tags: Properly manage meta tags for each page to enhance SEO.
- Dynamic Content: Handle dynamic content carefully to ensure it is indexed correctly.
Potential Drawbacks
SSR is not without its drawbacks. Some potential issues include:
- Increased Complexity: SSR adds complexity to the application architecture, requiring careful handling of server and client code.
- Longer Development Time: Implementing SSR can increase development time due to the need for additional configuration and optimization.
- Hydration Issues: Ensuring that the client-side JavaScript matches the server-rendered HTML can sometimes lead to hydration issues, where the client-side code fails to attach properly to the server-rendered content.
Best Practices
To effectively implement SSR, consider the following best practices:
- Code Splitting: Use code splitting to reduce the size of JavaScript bundles sent to the client.
- Data Fetching: Fetch data on the server before rendering to ensure the HTML sent to the client is complete.
- Error Handling: Implement robust error handling to manage potential issues during server-side rendering.
In conclusion, while SSR offers significant benefits in terms of performance and SEO, it also introduces challenges that must be carefully managed. By understanding these considerations and following best practices, you can optimize your SSR implementation for better performance and user experience.
Conclusion
Server-side rendering (SSR) in React offers a powerful approach to building fast, SEO-friendly web applications. By rendering content on the server, SSR provides faster initial page loads and improved search engine visibility, addressing key challenges in modern web development. Throughout this guide, we have explored the fundamentals of SSR in React, from its basic concepts to implementation details and key considerations. We have seen how SSR can significantly enhance user experience and application performance when implemented correctly.
Remember, while SSR offers many benefits, it is not a one-size-fits-all solution. Always consider your specific use case and requirements when deciding whether to implement SSR in your React projects. By mastering SSR in React, you are equipping yourself with a valuable skill that can significantly improve the quality and performance of your web applications. Keep learning, experimenting, and building!